Suggested Learn then move next.
1. Introduction.
In this Tutorial , we will discuss
1. Writing to the console
While working with Console applications in .NET, we use Console [ a Class in .net], which is contained inside System Namespace, as shown below by IntelliSense that class System.Console => represents the Standard input, Output and error streams for console applications. This class can't be inherited.

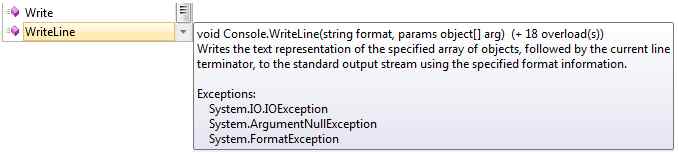
we will see the difference between the Write and the WriteLine methods.
OutPut:-OneTwoThree
Four
Reading to a Console
When we run a console application from the debugger, it automatically close the console window when the application ends, preventing you from seeing the output.
There are Three methods :
1. Console.Read()
is used to read the next character from the standard input stream. This method basically blocks its return when the user types some input characters. As soon as the user press ENTER key it terminates.(Simple word Reads just a single character)

Reads the next character from the standard input stream. it only accept single character from user input and return its ASCII Code.
Note: Data type should be int. because it return an integer value as ASCII.
It obtains the next character or function key pressed by the user.In Simple words.it read that which key is pressed by user and return its name.it does not require to press enter key before entering.
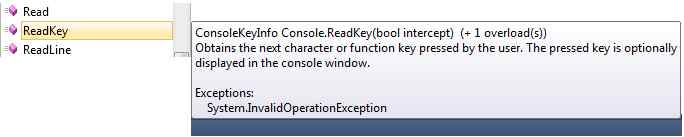
Reads the next line of characters from the standard input stream. simply you can say, it read all the characters from user input. (and finish when press enter)
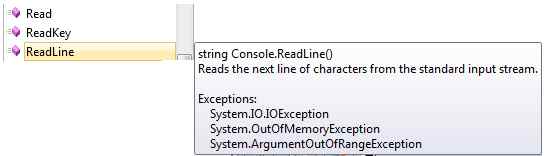
Example:-
When I Enter a String "Chandan Kumar Pandey" it read as it is.its mean it reads all character until the end of line.
HelloWorld
1. Introduction.
In this Tutorial , we will discuss
1. Writing to the console
a) Console.Write();
b) Console. WriteLine();
2. Reading from the consolea) Console.Read();
b) Console.ReadKey();
c) Console.ReadLine();
1. Writing to a Console
While working with Console applications in .NET, we use Console [ a Class in .net], which is contained inside System Namespace, as shown below by IntelliSense that class System.Console => represents the Standard input, Output and error streams for console applications. This class can't be inherited.
There are two methods for writing to the console, which are used extensively:
- Console.Write(): Write the specified value to the console window. Write() method has 17 overloads as shown below by intelliSense.

2. Console.WriteLine(): This is one of the output methods of the Console class in the run-time library. This does the same, but adds a newline character at the end of the output using specified format information. WriteLine() method has 18 overloads as shown below by IntelliSense.
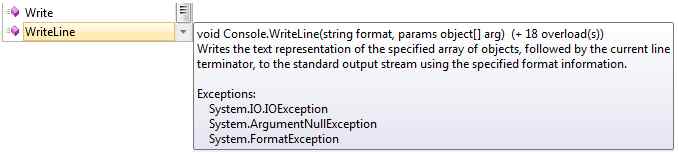
we will see the difference between the Write and the WriteLine methods.
The Write () method outputs one or more values to the screen without a new line character.
The WriteLine() always appends a new line character to the end of the string. this means any subsequent output will start on a new line.
using System;
class Program {
static void Main() {
Console.Write("One");
Console.Write("Two");
// this will set a new line for the next output
Console.WriteLine("Three");
Console.WriteLine("Four");
}
}
OutPut:-OneTwoThree
Four
Reading to a Console
When we run a console application from the debugger, it automatically close the console window when the application ends, preventing you from seeing the output.
There are Three methods :
1. Console.Read()
is used to read the next character from the standard input stream. This method basically blocks its return when the user types some input characters. As soon as the user press ENTER key it terminates.(Simple word Reads just a single character)

Reads the next character from the standard input stream. it only accept single character from user input and return its ASCII Code.
Note: Data type should be int. because it return an integer value as ASCII.
As I input "6" and it return its ASCII value "54.
2. Console.ReadKey()
It obtains the next character or function key pressed by the user.In Simple words.it read that which key is pressed by user and return its name.it does not require to press enter key before entering.
Note: it is STRUCT Data type which is ConsoleKeyInfo.
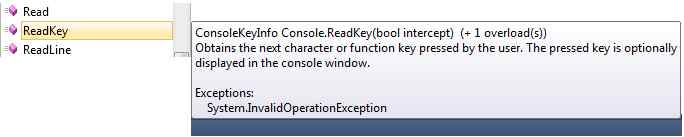
as I press different Buttons from keyboard like Escape,Tab,Enter,Spacebar, R, P it will return the name.
3. Console.ReadLine()
Reads the next line of characters from the standard input stream. simply you can say, it read all the characters from user input. (and finish when press enter)
Note: it return a STRING so data type should be STRING
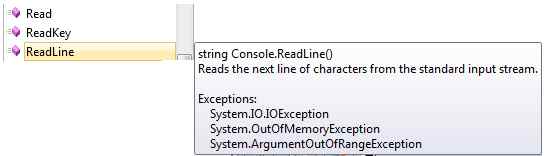
Example:-
When I Enter a String "Chandan Kumar Pandey" it read as it is.its mean it reads all character until the end of line.
Combining (Concatenating) two strings using + operator and printing them
Strings can be combined/concatenated using the
+
operator while printing.using System;
namespace Sample
{
class Test
{
public static void Main(string[] args)
{
int val = 55;
Console.WriteLine("Hello " + "World");
Console.WriteLine("Value = " + val);
}
}
}
When we run the program, the output will be--
HelloWorld
55
Printing concatenated string using Formatted String [Better Alternative]
A better alternative for printing concatenated string is using formatted string. Formatted string allows programmer to use placeholders for variables. For example,
The following line,
Console.WriteLine("Value = " + val);
can be replaced by,
Console.WriteLine("Value = {0}", val);
{0}
is the placeholder for variable val which will be replaced by value of val. Since only one variable is used so there is only one placeholder.
Multiple variables can be used in the formatted string. We will see that in the example below.
using System;
namespace Sample
{
class Test
{
public static void Main(string[] args)
{
int firstNumber = 5, secondNumber = 10, result;
result = firstNumber + secondNumber;
Console.WriteLine("{0} + {1} = {2}", firstNumber, secondNumber, result);
}
}
}
When we run the program, the output will be
5 + 10 = 15
Here,
{0}
is replaced by firstNumber, {1}
is replaced by secondNumber and {2}
is replaced by result. This approach of printing output is more readable and less error prone than using +
operator.
Let us see how to get user input from user and convert it to integer.
Firstly, read user input −
int a = Convert.ToInt32(val);
Console.WriteLine("Your input: {0}",a);
Syntax: public static int Read ();
Return Value: It returns the next character from the input stream, or a negative one (-1) if there are currently no more characters to be read.
Exception: This method will give IOException if an I/O error occurred.
Reference:
- https://docs.microsoft.com/en-us/dotnet/api/system.console.read?view=netframework-4.7.2
- Thanks for reading this article, hope you enjoyed it.If any Query drop a comment.