19 Arrays
we will discuss..
1. Array
2. Multidimensional Array
3. Jagged arrays
4. Passing arrays to function
5. Param arrays
Array:
An array are used to stores fixed-size number of element values of the same type. Array is used to store a collection of data same type stored at contiguous memory location, array index starts from 0. We can store only fixed set of elements in C# array.
Different ways Array Declaration and Initialization
To create an array of string, you could write:
1. string[]name="chandan","amit","biplob","prakash","gaurav","marry"};
To create an array of integers, you could write:
2. int [] num ={ 10, 14, 24, 34 }; or int[] num = new int[]{ 10, 14, 24, 34 };
3. int [] num = new int[5];//creating array
You cannot place square brackets after the identifier.
int arr[] = new int[5];//compile time error
4. string[] str1;
str1 = {"chandan","amit","biplob","prakash" }; // error : wrong initialization of an arrayMultidimensional Array:
multidimensional array is also called as rectangular arrays in C#. It can be support either two dimensional or three dimensional.Multidimensional Arrays can be declared by specifying the data type of an elements followed by the square brackets [] with comma (,) separator inside the square brackets..The data is stored in tabular form (row * column) which is also known as matrix. which has x number of row and y number of column.
Following way to creating two or three dimensional arrays in c# programming language.
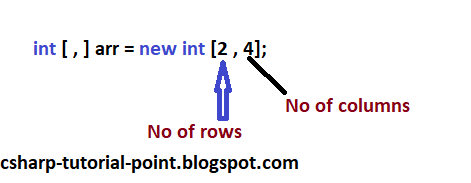
int[,] arr=new int[3,3];//declaration of 2D array
public class 2Darray
{
public static void Main(string[] args)
{
int [,] arr = new int [3,3]; //declaration of 2D array
arr [0,1] = 10; //initialization
arr [1,2] = 20;
arr [2,0] = 30;
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
Console.Write(arr[i,j]+" ");
}
Console.WriteLine(); //new line at each row
}
Console.ReadLine();
}
}
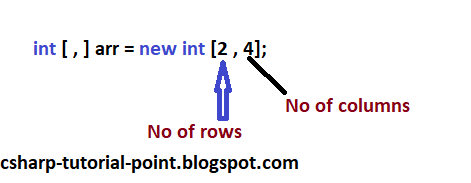
int[,] arr=new int[3,3];//declaration of 2D array
// Two-dimensional array.
1. int[,] arrayTwoD = new int[,] { { 1, 2 }, { 3, 4 }, { 5, 6 }, { 7, 8 } };
// The same array with dimensions specified.
2. int[,] arrayTwoDa = new int[4, 2] { { 1, 2 }, { 3, 4 }, { 5, 6 }, { 7, 8 } };
// A similar array with string elements.
3. string[,] arrayTwoDb = new string[3, 2] { { "one", "two" }, { "three", "four" },
{ "five", "six" } };
{
public static void Main(string[] args)
{
int [,] arr = new int [3,3]; //declaration of 2D array
arr [0,1] = 10; //initialization
arr [1,2] = 20;
arr [2,0] = 30;
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
Console.Write(arr[i,j]+" ");
}
Console.WriteLine(); //new line at each row
}
Console.ReadLine();
}
}
Output
0 10 0
0 0 20
30 0 0
int[,,] arr=new int[3,3,3];//declaration of 3D array
public class 2Darray{public static void Main(string[] args){int [, ] arr = { { 10, 20, 30 }, { 40, 50, 60 }, { 70, 80, 90 } };//declaration and initialization
for(int i=0;i<3;i++){for(int j=0;j<3;j++){Console.Write(arr[i, j]+" ");}Console.WriteLine(); //new line at each row}Console.ReadLine();}}Output
10 20 30
40 50 60
70 80 90
How can you declare array ?
int[ , ] arr;
arr = new int[ , ] { { 1, 2}, {3, 4}, {5, 6} }; //success
arr = {{1,2}, {3,4},{4,5},{6,7}}; //Error